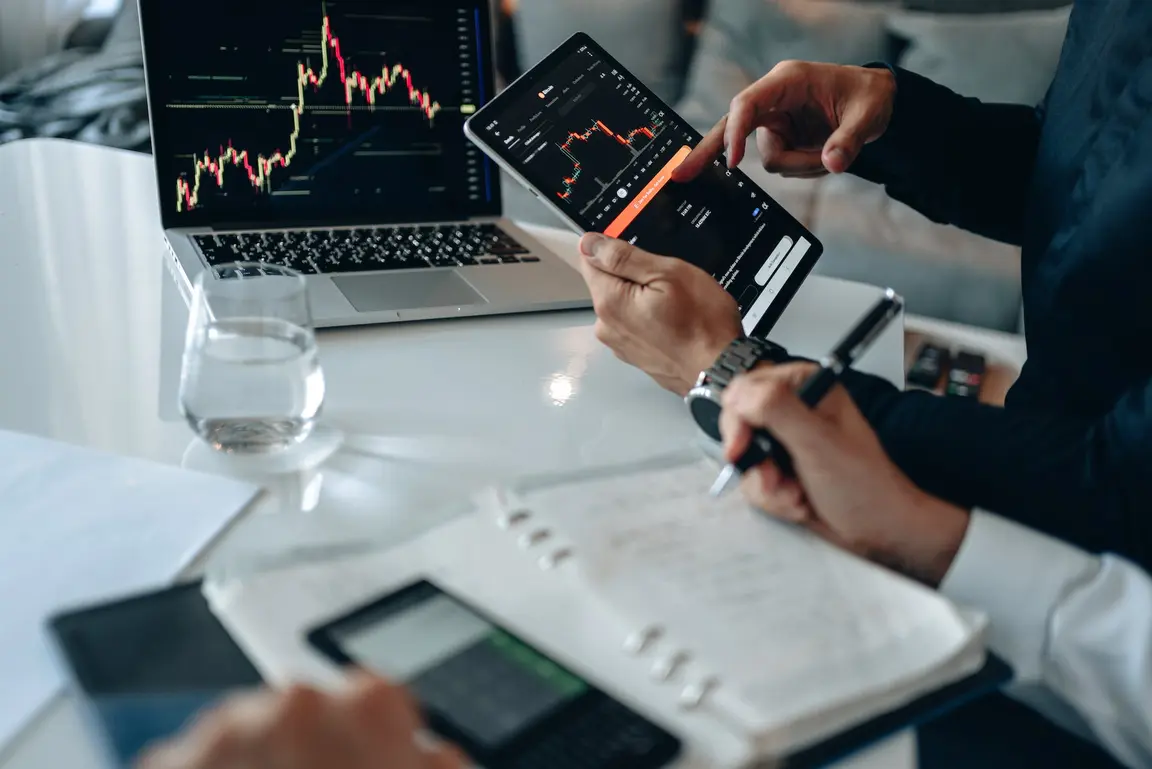
Enhancing WebSocket Scalability with a Python Proxy
30 November 2023
WebSocket Protocol has transformed real-time communication on the web, enabling bidirectional interaction between clients and servers. It works by implementing HTTP protocol and using a connection upgrade to establish a WebSocket connection. However, as your application grows, scalability challenges may arise. This blog post will explore the use of a WebSocket proxy server in Python to efficiently manage and distribute data, ensuring optimal performance and scalability.
The Challenge of Scaling WebSocket Applications
WebSocket applications often face hurdles when handling a large number of concurrent connections. A single WebSocket server may struggle to efficiently manage and respond to an increasing number of clients. This is where a WebSocket proxy becomes an invaluable tool.
Understanding WebSocket Proxies
A WebSocket proxy serves as an intermediary between clients and servers, overseeing the flow of WebSocket traffic. It manages the initial WebSocket handshake, distributes incoming connections, and forwards WebSocket frames between clients and the actual WebSocket server.
Scenario: Single WebSocket Source
Consider having a WebSocket source generating real-time data, such as a financial market feed or a live chat system. The objective is to distribute this stream effectively to a growing number of clients without overwhelming the original WebSocket server.
Implementation with a Python WebSocket Proxy
WebSocket Handling:
1. Initial Handshake:
When a client initiates a WebSocket connection, it communicates with the WebSocket proxy. The proxy performs the initial handshake and establishes a connection.
2. Load Distribution:
The WebSocket proxy intelligently distributes incoming connections among multiple instances of the original WebSocket server. This ensures an even workload distribution, preventing any single server from becoming a bottleneck.
3. Efficient Forwarding:
As WebSocket frames arrive from clients, the proxy efficiently forwards them to the appropriate WebSocket server instance. This allows for parallel processing and improves the application's overall responsiveness.
4. Aggregated Responses:
The proxy collects responses from WebSocket server instances and relays them back to clients. Clients interact with the proxy, unaware of the behind-the-scenes distribution.
Python Implementation:
To implement a WebSocket proxy in Python, you can use libraries like "websockets" and "asyncio". Please refer to our tutorial on Scaling WebSocket with Python for full code.
Advantages of WebSocket Proxies in Python
1. Scalability:
WebSocket proxies enable horizontal scaling, allowing your application to handle a larger number of clients seamlessly.
2. Load Balancing:
WebSocket proxies can incorporate load-balancing algorithms, ensuring an intelligent distribution of connections to prevent server performance bottlenecks.
3. Fault Tolerance:
In case of a WebSocket server instance failure, a WebSocket proxy can reroute connections to healthy instances, ensuring continuous service.
Conclusion
Scaling WebSocket applications can be achieved seamlessly with the introduction of a WebSocket proxy, especially when implemented in Python using libraries like "websockets" and "asyncio". Whether managing financial data, live chats, or any other WebSocket-driven application, a Python WebSocket proxy can be the key to unlocking scalability and performance.