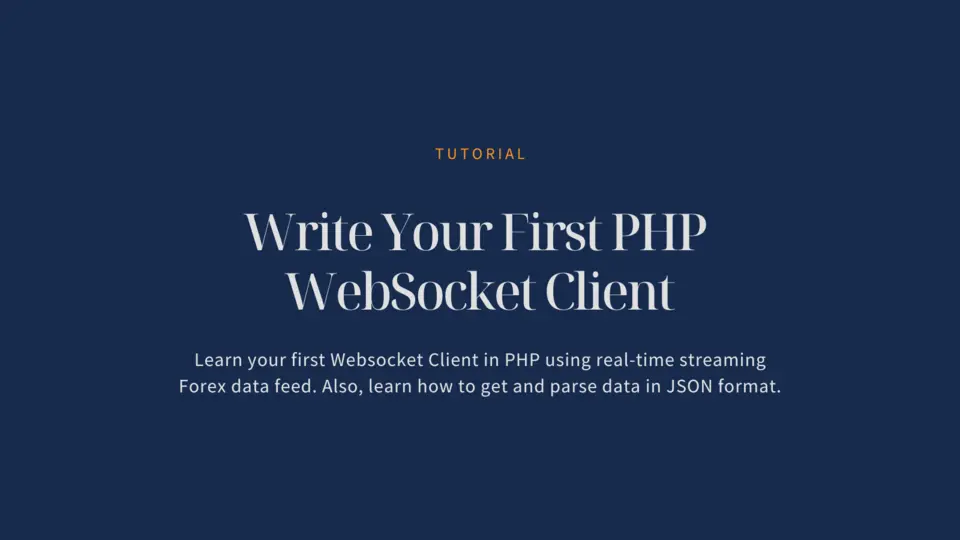
Your First PHP WebSocket Client
29 April 2022
This tutorial is designed to get you started with WebSocket in PHP and show you how to receive real-time market data. WebSockets provide a two-way, full-duplex communication channel that functions over an HTTP connection, unlike HTTP requests, which are unidirectional and stateless. We will use a simple editor and Composer package manager to make downloading the required packages simple.
Setup the Environment
For this tutorial, you will need to have PHP installed; you can check if you already have it installed by opening command windows and typing "php.exe -version" If you get a version number output, you can skip to the coding section. If not, you will need to install PHP. You will also need an API key for the Forex data service. You can sign up for Free and get your streaming API key by starting a 14-day WebSocket trial from your user dashboard or a pre-populated code sample from our data docs page.
Setup PHP
Setting up PHP is very simple and can be done in 3 easy steps.
1) Download the build file that contains php.exe from the PHP site and unpack the archive into a new directory.
2) Update your "Environment Variables" or classpath to invoice the location of PHP.exe.
3) To verify your setup, load a command window and run the following command, the output will be the version number of your PHP install.
//for windows php.exe -version // for linux php -version
expected output.
PHP 7.2.24-0ubuntu0.18.04.13 (cli) (built: Jul 6 2022 12:23:22) ( NTS ) Copyright (c) 1997-2018 The PHP Group Zend Engine v3.2.0, Copyright (c) 1998-2018 Zend Technologies with Zend OPcache v7.2.24-0ubuntu0.18.04.13, Copyright (c) 1999-2018, by Zend Technologies
Setup the project directory
Let's get started by creating a directory to store our source code and dependencies. I have called this directory WebScoketClientPHP, but you can call it anything you wish.
Now, we need to install the Composer package manager. Once this has been established, we can download the package we need. Go to the directory created in the previous step and run the following command.
composer require textalk/websocket
Let's write some code
In your source directory create a new file called WebSocketClient.php and open this with your favorite development environment of text editor.
First, we will add the PHP tags and our require_once statement to import our packages from Composer.
<?php require_once("vendor/autoload.php"); ?>
Now, we can create a new WebSocket connection by passing in the URL for the WebSocket service, as shown below. We assign this new WebSocket to a variable client.
$client = new WebSocketClient("wss://marketdata.tradermade.com/feedadv");
The WebSocket sends a "connected" message to the WebSocket server, so we need to listen to this and then send our login response with our API Key.
$message = $client->receive(); echo $message; $client->text("{"userKey":"YOUR_API_KEY", "symbol":"GBPUSD,EURUSD"}");
Now the connection is established and we will now enter into a loop of receiving live exchange rates.
while(true){ $message = $client->receive(); echo " Data ", $message, " "; }
We can run our program and look out at the output
php WebSocketClient.php
Voila! We are now streaming live forex rates.
Data {"symbol":"GBPUSD","ts":"1651244018768","bid":1.25486,"ask":1.25486,"mid":1.25486} Data {"symbol":"GBPUSD","ts":"1651244018790","bid":1.25486,"ask":1.25488,"mid":1.2548699} Data {"symbol":"GBPUSD","ts":"1651244018791","bid":1.25482,"ask":1.25488,"mid":1.2548499} Data {"symbol":"GBPUSD","ts":"1651244018796","bid":1.25487,"ask":1.25488,"mid":1.254875} Data {"symbol":"GBPUSD","ts":"1651244018815","bid":1.25485,"ask":1.25488,"mid":1.2548649} Data {"symbol":"GBPUSD","ts":"1651244018816","bid":1.25485,"ask":1.25486,"mid":1.254855} Data {"symbol":"GBPUSD","ts":"1651244018842","bid":1.25485,"ask":1.25487,"mid":1.25486} Data {"symbol":"GBPUSD","ts":"1651244018866","bid":1.25485,"ask":1.25486,"mid":1.254855} Data {"symbol":"GBPUSD","ts":"1651244018868","bid":1.25484,"ask":1.25486,"mid":1.25485}
Now that we have the data we can look at parsing this into a usable format. First, we need to check if the data received is the "connected" message or a data item. Then we can parse the data and extract the elements.
if(strcmp($message,"connected") !== 0){ $decoded_json = json_decode($message); echo $decoded_json->symbol, $decode-json->ts, $decode_jdon->bid, $decode__json->ask, " "; }
You should now see the currency exchange rates parsed in real-time.
EURUSD 1651244529366 1.05515 1.05517 GBPUSD 1651244529371 1.2554 1.2554 GBPUSD 1651244529385 1.2554 1.25541 EURUSD 1651244529389 1.05515 1.05516 EURUSD 1651244529416 1.05518 1.05519 GBPUSD 1651244529455 1.25542 1.25543 GBPUSD 1651244529458 1.25542 1.25546 GBPUSD 1651244529492 1.2554 1.25546 GBPUSD 1651244529496 1.2554 1.25545
We are done. You should now have a working WebSocket.WebSockets provide a duplex communication channel, which means they allow the sending and receiving of data over a TCP connection. If you have any questions, please don't hesitate to get in touch via our contact page or our online chat.